Do you use the hibernation feature of Windows? Ever had any problems with it? I suspect that the answers to these two questions generally are the same…
Firstly, if you’re using Windows XP and have more than 2 GB of RAM, it doesn’t work. Fortunately there’s a fix. For more info, see here.
Secondly, you’re likely to have run into a host of other problems. My experiences include:
- Hibernation works, but the computer is immediately awakened
- Instead of hibernating, the computer is turned off
- Instead of hibernating, I’m logged out to the welcome screen
All these are extremely irritating, of course. Fortunately I’ve found a solution to all my problems so far, namely a little known but excellent free tool called MCE Standby tool (MST) that helps you configure the hibernation options. If you have similar problems to mine, give it a go!
When you install the tool, it puts a small, green “power” icon in the system tray:
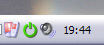
To configure the hibernation options, right-click the system tray icon, and the main window is displayed:
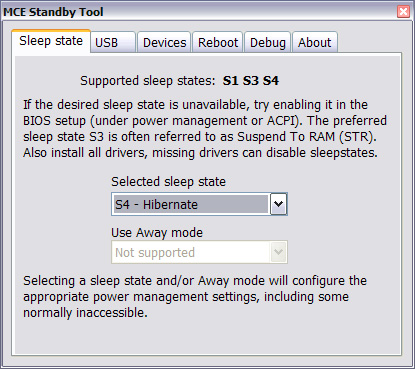
To fix my two last problems above, I changed Selected sleep state to “S3”, restarted the computer (might be unnecessary) and then changed back to “S4”. Voilá, problems gone.
If you have problems with the computer awakening immediately after hibernation then it might be a USB-connected device that’s waking the computer up (a mouse, keyboard, remote control receiver, etc). To fix this, you can select which deviced should be allowed to wake the computer up and this is done in the Devices tab:
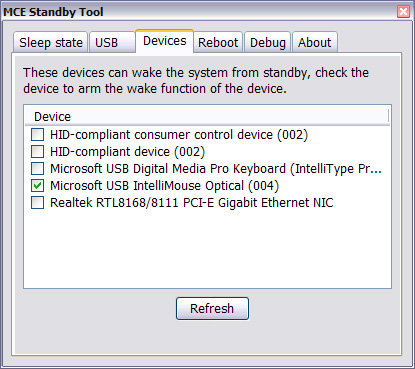
Deselect all devices you suspect to be causing problems, and no awakening should occur. For me it was my keyboard.
There are more options in the useful little tool, but these are the ones that helped me so far. Give it a try if you have similar problems!
/Emil
BTW, “MCE” in the tools name stands for “Media Center Edition” indicating that hibernation problems can often be related to media systems. And indeed, many of my problems started after I installed Media Portal. My advice concerning that system is of course to keep away from it…